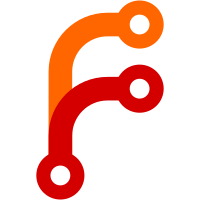
That makes it easier to identify "license only" headers (because they are now license only) Script line used for that: perl -i -p0e 's|/\*.*\n.*This file is part of the coreboot project.*\n.*\*|/* This file is part of the coreboot project. */\n/*|' # ...filelist... Change-Id: I2280b19972e37c36d8c67a67e0320296567fa4f6 Signed-off-by: Patrick Georgi <pgeorgi@google.com> Reviewed-on: https://review.coreboot.org/c/coreboot/+/41065 Tested-by: build bot (Jenkins) <no-reply@coreboot.org> Reviewed-by: David Hendricks <david.hendricks@gmail.com> Reviewed-by: HAOUAS Elyes <ehaouas@noos.fr> Reviewed-by: Paul Menzel <paulepanter@users.sourceforge.net> Reviewed-by: Angel Pons <th3fanbus@gmail.com>
113 lines
2.9 KiB
C
113 lines
2.9 KiB
C
/* This file is part of the coreboot project. */
|
|
/*
|
|
* Copyright (C) 2016 Google Inc
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; version 2 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include <pci/pci.h>
|
|
#include "pci-userspace.h"
|
|
|
|
#define DEBUG_PCI 1
|
|
|
|
static struct pci_access *pacc;
|
|
|
|
int pci_initialize(void)
|
|
{
|
|
struct pci_dev *dev;
|
|
|
|
pacc = pci_alloc();
|
|
|
|
pci_init(pacc);
|
|
pci_scan_bus(pacc);
|
|
for (dev = pacc->devices; dev; dev = dev->next) {
|
|
pci_fill_info(dev, PCI_FILL_IDENT | PCI_FILL_BASES);
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
int pci_exit(void)
|
|
{
|
|
pci_cleanup(pacc);
|
|
return 0;
|
|
}
|
|
|
|
u8 pci_read_config8(struct device *dev, unsigned int where)
|
|
{
|
|
struct pci_dev *d;
|
|
if ((d = pci_get_dev(pacc, 0, dev->busno, dev->slot, dev->func)))
|
|
return pci_read_byte(d, where);
|
|
#ifdef DEBUG_PCI
|
|
printf("PCI: device not found while read byte (%x:%x.%x)\n",
|
|
dev->busno, dev->slot, dev->func);
|
|
#endif
|
|
return 0;
|
|
}
|
|
|
|
u16 pci_read_config16(struct device *dev, unsigned int where)
|
|
{
|
|
struct pci_dev *d;
|
|
if ((d = pci_get_dev(pacc, 0, dev->busno, dev->slot, dev->func)))
|
|
return pci_read_word(d, where);
|
|
#ifdef DEBUG_PCI
|
|
printf("PCI: device not found while read word (%x:%x.%x)\n",
|
|
dev->busno, dev->slot, dev->func);
|
|
#endif
|
|
return 0;
|
|
}
|
|
|
|
u32 pci_read_config32(struct device *dev, unsigned int where)
|
|
{
|
|
struct pci_dev *d;
|
|
if ((d = pci_get_dev(pacc, 0, dev->busno, dev->slot, dev->func)))
|
|
return pci_read_long(d, where);
|
|
#ifdef DEBUG_PCI
|
|
printf("PCI: device not found while read dword (%x:%x.%x)\n",
|
|
dev->busno, dev->slot, dev->func);
|
|
#endif
|
|
return 0;
|
|
}
|
|
|
|
void pci_write_config8(struct device *dev, unsigned int where, u8 val)
|
|
{
|
|
struct pci_dev *d;
|
|
if ((d = pci_get_dev(pacc, 0, dev->busno, dev->slot, dev->func)))
|
|
pci_write_byte(d, where, val);
|
|
#ifdef DEBUG_PCI
|
|
else
|
|
printf("PCI: device not found while write byte (%x:%x.%x)\n",
|
|
dev->busno, dev->slot, dev->func);
|
|
#endif
|
|
}
|
|
|
|
void pci_write_config16(struct device *dev, unsigned int where, u16 val)
|
|
{
|
|
struct pci_dev *d;
|
|
if ((d = pci_get_dev(pacc, 0, dev->busno, dev->slot, dev->func)))
|
|
pci_write_word(d, where, val);
|
|
#ifdef DEBUG_PCI
|
|
else
|
|
printf("PCI: device not found while write word (%x:%x.%x)\n",
|
|
dev->busno, dev->slot, dev->func);
|
|
#endif
|
|
}
|
|
|
|
void pci_write_config32(struct device *dev, unsigned int where, u32 val)
|
|
{
|
|
struct pci_dev *d;
|
|
if ((d = pci_get_dev(pacc, 0, dev->busno, dev->slot, dev->func)))
|
|
pci_write_long(d, where, val);
|
|
#ifdef DEBUG_PCI
|
|
else
|
|
printf("PCI: device not found while write dword (%x:%x.%x)\n",
|
|
dev->busno, dev->slot, dev->func);
|
|
#endif
|
|
}
|